Zdepth is a Normalized variable. Normalized means between 0-1, as a real(float).
Normalizing is a very good thing, it allows you to really simplify the math, especially when what you want is a relationship between two things, rather than a solid number.
So in this case, you'll be normalizing the y coordinate.
if its a 640x480 game, with screen center being 0 (default), you'd normalize Y like this:
normy=(actor.y+240)/480;
where normy is a real variable. This will return a real(float) between 0 and 1.
Fuzzy showed me another awesome trick(not really needed in ge since Mak gave us distance and direction functions) but cool anyway.
This uses 2 functions - normalize and speedmulti. (functions are at the bottom)
variables (all real):
lookx; - the target coordinates
looky;
offsetx; -the difference between x and targetx
offsety;
speed; - the actor's speed
- Code: Select all
lookx=targetactor.x; //set the target coordinates each time (because they'll get normalized!)
looky=targetactor.y; //targetactor refers to whatever your actor is going to move toward.
offsetx=lookx-x; //here we find the distance between actor.x and target.x
offsety=looky-y; //same for y
normalize(offsetx, offsety); //convert the offsets to normalized floats.
//now we have the ratio of x to y.
speedmulti(offsetx, offsety);//convert it to the speed of the actor
x+=offsetx; //add the final results to the current xy position.
y+=offsety;
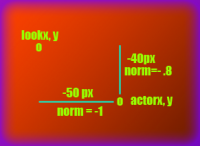
- In this case, if your speed was 8, you'd move x-8, y-6.4.
Please note that these functions are not really usable because we haven't established the in/out methods for the numbers - how you pull out actor variables and put them back in is up to you. You can use a float function and return the float, or you can use offsetx[cloneindex] and not have to return anything, or whatever you like. I might have written this right into the script instead of making functions, but you can reuse functions. As you make more and more games, you're gonna get tired of starting from scratch every time. You want to write scripts you can reuse and easily implement in future games.- Code: Select all
void normalize(float xx, float yy){
if(xx>yy){
offsetx=xx/abs(xx); //the larger number becomes 1. We use this equation to preserve the +- of the number.
offsety=(yy/xx); //the smaller number is the ratio of the two numbers.
}
else if(yy>xx){
offsetx=yy/abs(yy);
offsety=(xx/yy);
}
- Code: Select all
void speedmulti(float xx, float yy){
offsetx=xx*speed; //this one's pretty simple.
offsety=yy*speed;
}
This will allow a player to move toward an object without using the direction(x, y, x, y); function. Not all that useful in ge, but a good example of how powerful normalizing can be.
Thus ends your introduction to normalizing. Only bother reading the rest of this if you're really interested in beefing up your game speed and make more complex actor interaction.
A second normalizing scenario would be a mass of units with lots of specific variables, as in an rts game. If i cast a mass slow spell, which targets all your enemy's units, then everyone's speed is reduced by a certain amount; You could use a simple decimal for that, like this:
- Code: Select all
myspeed=speed;
speed*=slowspellamount; //like .8, for instance.
and when the slowspell wears off, return speed to normal.
- Code: Select all
speed=myspeed;
The problem occurs when you decide to cast 2 slow spells, 1 freeze spell, and a poison spell on those enemies all at once.
First, the second slow spell will reduce the speed in reductive squares - that is, it will multiply the already reduced speed by .8. This means that the more slow spells you cast at once, the less effective each one is (which may be what you want, but anyway). And the ice spell won't do jack if they've already got a bunch of slow spells on them, because after 4 slow spells at .8, a speed of 10 becomes a speed of four. The ice spell, as a decimal itself, won't do much slowing. This means that if you cast 100 slow spells on an enemy, he will still have a speed! That doesn't seem right does it? 100 slow spells should definitely make the actor stop cold!
You can't use solid reductions - if the slow spell reduces speed by 2, then you can easily end up with negative speeds - we don't want negative values for unit attributes, this would be very bad! And while you can insert an 'if (speed<0){speed=0;}',
if you add lots of bandaids like this, when you have 220 space marines doing it you end up wasting a lot of cpu!
And this points out the issue here - speed, damage, health, these are all actual values. They can range anywhere from 0 to infinity. Now to avoid negatives, we use decimals (ratios) instead of actual values for the effects - now you have decimals interacting with real integers. From this point of view, you can see how nice it would be to have functions which interact with compatability.
Not just to keep the numbers safe, but also to make their interaction easier to understand, you normalize all of the numbers before computing their interactions.
If you've set up a system of normalization for the interactions between effects and variables, you can keep a tight grip on the math, and avoid unpredictable numbers.
Lets say poison reduces the enemy health by 10%;
an enemy with 100 health, after you cast 10 poison spells on him, will have a health of 34, not 0.
Now i'll end this post with fifty biased questions:
If you wanted 10 10% poison spells to kill the enemy, how would you go about it?
Here's some suggestions:
1. add the poison spells together first, then use that percentage - 10 x 10% =100% = death.
//but what will happen if i cast nine then wait a second before casting the 10th?
2. add the poison spells as a cumulative number -store the total poison in the body and use that as a calculation.
//but how will you dump these? //how will you manage lots of different stored spell effects, in tons of different actors?
//and will you have to run the equation every single time for every single actor?
3. calculate the force of the combined units as a whole, calculate the poisoning only
once - then simply send the damage to all the enemies?

//how will you compensate for the varying degrees of poison resistance between the different units without yet again writing another equation to handle the damage as it comes in?
//hint:
normalizingComments, questions, snyde remarks all welcome.