Even nine actors are overkill. This is a job that would work well with just one canvas to draw on, one canvas to show the board lines, and maybe 2 actors to store the X and O shapes. But you could draw them too.
I would make a small nine element array. I wouldnt make it a 2d array even. In theory you dont even need this.
- Code: Select all
char squares[9];
Let a blank equal 0, an X equal 1 and a O equal 5 in the array. That is, when the player clicks a square, fill in a 5 at that array point if he is playing with O.
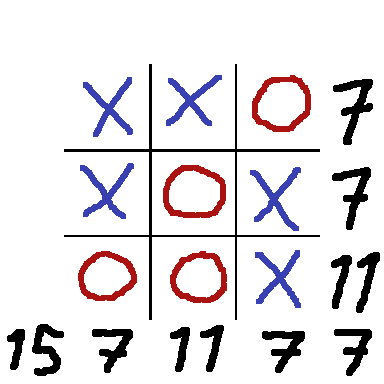
As the blanks are filled in, store the values of rows, columns and diagonals in a array variable.
- Code: Select all
int scores[9]; // I use nine, the last value tracks if the game is over.
The reason for this is that it doesn't make much sense to continuously add all of them up. Do it once and be done with it. Otherwise its making the computer work for no reason. Sure, its not a lot of work, but the philosophy counts very much on bigger programs.
The reason for the values I chose were simple. They are prime numbers and in the space that you have, three X's can never add up to a value that can be mistaken for one O. This is called meaningful data. It means something to the programmer, and its also easy to make it mean something to the AI. A bunch of if statements is vague.
Now all you have to do is check each row and compare its value against 3 and 15. if either is the result, that tells which player has won.
As an aside, the word information comes from this technique. Our possible formations exclude the possibility of data collisions. That is, there are no ambiguous results. We can also refer to this system as scoring, and it will allow the AI to decide where the next best move is.
if the row/column score is 3 or 15, somebody won.
If the row/column score is 7 or 11, then the computer may not use that row. It is full.
Since players take turns, and X always goes first, the O can only ever have 4 squares, and the score has to be 25 at the end of the game. so...
- Code: Select all
if (scores[9] == 25) { game over();}