But the only rotation possible since now is either integrated only in gE1.4.2
or.. my project BitFox. BitFox (Library for image manipulation) can rotate a bitmap from gE and foxyGI (library for interface widgets) can render it.
You aware of that?
Okay, I approve your request. The imminent tutorial will contain:- Splay Acceleration (rotation)
- And eventually (Although it is about to become too broad) - Contractility Rope Physics
Needed equipment:Best usage:Side-view car games, bounces etc
ETA:-----
___________________________________________________________________________________________________________________________________
Let's start with the Splay Acceleration.
If some arbitrary entity (load) gets onto a given inclined plane, depending on the spline (inclination and surface shape (friction and resistance (vertical for gravity and horizontal for opposing force)), depending on entity's shape (friction and resistance (vertical for gravity/weight and horizontal for opposing force), the entity (load) will commit movement in the direction of the slope (videlicet inertia), including rotation (the act of gravity).
Evident in everything, a lot of forces play an important role in all this and so as the name suggests, it will involve an arcane amount of physical formulas, but it's okay, just bear with me and we will programmatically simulate advanced prime physics from the scratch, in Game-Editor. Let's get started.
1. Creating forcesStart Game-Editor and add several Actor variables by virtue of the Game-Editor's Variable Declarations Pane nearby any Script Editor.

Add these variables, all of them should become from "Integers" to "Real" and from "Global" to "Actor variable"
- gravity
- friction
- inclination
2. Creating entitiesWe need to create two test functions that can render on-canvas our load and the inclined plane.
Their shape will be configurable so we can test any interaction.
And for that matter, the function for creating our load must be based on trigonometry.
So we can have everything between circle (minimum friction) and a square (maximum friction * size) (wheres inbetween lies the hexagon).
This is called n-polygon, where
n determines the number of vertex (angles on degree).
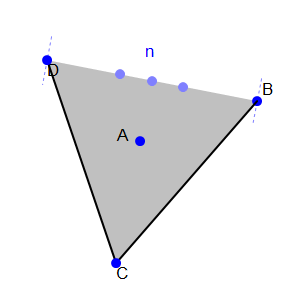
Again, this is only for test purposes, aside this tutorial you will be using any actor of your desire.
So I created this function:
- Code: Select all
void polygon (int n)
{
double pI = 3.141592653589;
double area = min(width / 2, height / 2);
int X = 0, Y = area - 1;
double offset = Y;
int lastx, lasty;
while(Y-->0)
{
double radius = sqrt(X * X + Y * Y);
double quadrant = atan2(Y, X);
int i;
for (i = 1; i <= n; i++)
{
double interval = i * 255 / n;
lastx = X; lasty = Y;
quadrant = quadrant + pI * 2.0 / n;
X = round((double)radius * cos(quadrant));
Y = round((double)radius * sin(quadrant));
setpen(interval, interval, interval, 0.0, 2);
moveto(offset + lastx, offset + lasty);
lineto(offset + X, offset + Y);
}
}
}
It accepts one argument, which is
n or the number of vertexes (edges / angles on degree). It draws a filled polygon with the sizes of the canvas
and fills it with interval color to make it look more fancy and a bit of 3D (Although it resembles cone on top view).

Anyway, since we will perform rotation on it, we need to change this function to draw the shape on a valid fgi bitmap instead.
It is very easy, all we have to do is specify the source argument and change the names of the drawing functions. The end code will look more like this:
- Code: Select all
void polygon (BYTE* buffer, int n)
{
double pI = 3.141592653589;
double area = min(width / 2, height / 2);
int X = 0, Y = area - 1;
double offset = Y;
int lastx, lasty;
while(Y-->0)
{
double radius = sqrt(X * X + Y * Y);
double quadrant = atan2(Y, X);
int i;
for (i = 1; i <= n; i++)
{
double interval = i * 255 / n;
lastx = X; lasty = Y;
quadrant = quadrant + pI * 2.0 / n;
X = round((double)radius * cos(quadrant));
Y = round((double)radius * sin(quadrant));
bitfox_setpen(interval, interval, interval, 0.0, 2);
bitfox_moveto(offset + lastx, offset + lasty);
bitfox_lineto_data(buffer, offset + X, offset + Y);
}
}
}
Paste this function in global code, follow the instructions of how to do load BitFox and how to load script in global code along it from the BitFox man page.
Warning: This function isn't intended to be drawn on each frame or multiple times as you may conclude from the GIF.
This function is highly non-optimized and uses rather destructive and inefficient way of filling.
We are just fine with it, since it will be created only on startup, but if you want a highly-optimized function to draw filled n-polygon, use
this instead. It is about 20 times faster.
Enough for the test code, create two canvas actors.
The smaller one with name
"load" and the other
"surface". Place and resize them according to this picture:
Now navigate to "load" Actor Control --> Create Actor --> Script Editor and paste these lines:- Code: Select all
erase(1, 1, 1, 1); // erases canvas of unused space
bitfox_create_data(width, height); // creates and inbuffers a valid bitmap image with the sizes of the canvas
bitfox_render_polygon(BMPDATA[0], 30); // uses our filled polygon function to draw a filled n-polygon in the newly created buffered bitmap image
// Note, BitFox will also render the drawing simultaneously on-canvas since it defines the [i]PRE_RENDERING[/i] macro, that defaults to [i]true[/i]
// and specifies whether this should happen or not
You can of course change the number of vertexes, 30 is just some random number enough to create a circle.
Change to 3 for triangle
Change to 4 for quadrangle
Change to 5 for pentagon
Change to 6 for hexagon
Change to 7 for septangle (very rare and ancient figure used in ancient Atlantis
Change to 8 for octagon
..you get the point

Now we need a simple mechanism to control the inclination of our surface.
Navigate to "surface" Actor Control --> Draw Actor --> Script Editor and paste these lines of code:- Code: Select all
char *key = GetKeyState();
static double tilt = 200, rightAcc, leftAcc;
int size = width - 9;
if(rightAcc > 0.0 || leftAcc > 0.0) // Condition to prevent of unnecessarily execution
{
setpen(64, 64, 64, 0.0, 16); // 16
erase(1, 1, 1, 1);
moveto(size - width, tilt);
lineto(size, (height - tilt));
}
if(key[KEY_LEFT])
{
if(rightAcc < 3.0)
{
rightAcc += .01;
tilt += rightAcc;
}
}
else
{
if(rightAcc > 0.0)
{
rightAcc -= .01;
tilt -= rightAcc;
}
}
if(key[KEY_RIGHT])
{
if(leftAcc < 3.0)
{
leftAcc += .01;
tilt -= leftAcc;
}
}
else
{
if(leftAcc > 0.0)
{
leftAcc -= .01;
tilt += leftAcc;
}
}
This clearly imitates scales or imaginary surface which inclination depends on the user key press.
TO BE CONTINUED