animpos=angle/90;
or instead of 90, use 360/nframes, for example, if you had 8 frames, it would be angle/45; but you can just use 360/nframes, as nframes is a builtin ge variable.
animpos=angle/(360/nframes);
As far as sin and cos, these are basic trigonometry functions which once you understand them, you're gonna love them.
In trig, we use radians for angles instead of degrees. Radians are an expression of PI (3.1459 etc). So we use
degtorad(angle) to convert to radians.
Then....cos = x and sin =y. If you were to make an angled line, you'd move over x pixels and up y pixels; a 45 degree angle is 1:1, and a 30 degree angle is 2:1. That's 2 x pixels for every y pixel, right? 90 is 0:1. x+=0, y+=1;
The last thing to know is that sin and cos return normalized values (a float less than 1) so you have to multiply them to get the distance you want, in this case, 20.
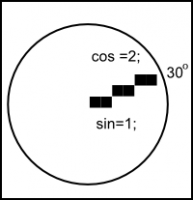
- This isn't technically correct; as sin and cos are floats less than 1; but the ratio is what i'm trying to show
So the sin/cos functions i wrote in the demo put the object in front of the user no matter which way he's facing, @ 20 pixels out from the player. When the player moves up and has angle of 90, the cos(x) =0, so it's centered on x, and when he's facing left or right, the sin(y)=0 so it's at the same y.
Actor * holds a temporary copy of an actor; ANY ACTOR IN THE GAME. You have to give it a name to refer to it, and then use either getclone or getclone2 to define it. (In global code, load the getclone2 script, hit file>save> and save it as a .c file so you can load it on other games). Getclone2 allows us to specify a cloneindex for the object, which in this demo, we got when we collided with the object.
so Actor*this=getclone2("object", oindex); Makes a temporary copy of the object we touched; you can then access this objects variables using the -> function; if oindex==10 then it will get object.10.
this->x; this->y; this->transp;
And it's the same for assigning or reading values, like:
this->x=100;
x=this->x;
For added functionality, you can use it alongside createactor, using createactor instead of getclone when creating a new actor:
Actor*this;
this=CreateActor("shot", "redmissile", etc. etc);
this->xvelocity=10;
at the end of the current script, Actor * this will disappear from the script, but the createactor version is now in the game as a normal actor.